Scanner.js JavaScript Scan in Browsers: Chrome, Edge, Firefox, IE
Scan Documents from TWAIN WIA scanners in Browsers and Upload to Server (Java, C# ASP.NET, PHP, Python, Ruby)
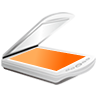
Scanner.js Quick Start
- The source code of the below tutorial can be viewed on Github;
- Please refer to the developer's guide for complete reference.
1. Include scanner.js to your HTML document
<script type="text/javascript" src="//cdn.asprise.com/scannerjs/scanner.js"></script>
Alternatively, you can host scanner.js and its required files on you owner servers.
2. Initiate A Scan
To initiate a scan, you can simply call scanner.scan with proper arguments. For example, the code below initiates a scan that will output images in jpg format:
function scanToJpg() { scanner.scan(displayImagesOnPage, { "output_settings" : [ { "type" : "return-base64", "format" : "jpg" } ] } ); }
You may use a button to invoke the above function:
<button type="button" onclick="scanToJpg();">Scan</button> <div id="images"/>
The div with name images will be used to display images scanned later. Once the user presses the Scan button, the scan dialog will present and the user can then scan documents. After the scanning is done, the result handler callback function will be invoked.
3. Handle The Scan Result Using A Callback Function
/** Processes the scan result */ function displayImagesOnPage(successful, mesg, response) { if(!successful) { // On error console.error('Failed: ' + mesg); return; } if(successful && mesg != null && mesg.toLowerCase().indexOf('user cancel') >= 0) { // User canceled. console.info('User canceled'); return; } var scannedImages = scanner.getScannedImage(response, true, false); // returns an array of ScannedImage for(var i = 0; (scannedImages instanceof Array) && i < scannedImages.length; i++) { var scannedImage = scannedImages[i]; processScannedImage(scannedImage); } } /** Images scanned so far. */ var imagesScanned = []; /** Processes a ScannedImage */ function processScannedImage(scannedImage) { imagesScanned.push(scannedImage); var elementImg = createDomElementFromModel( { 'name': 'img', 'attributes': { 'class': 'scanned', 'src': scannedImage.src } }); document.getElementById('images').appendChild(elementImg); }
First, we check whether the scan is successful or not. If successful, we check whether the user cancels - in which case no image will be returned.
The scanner.getScannedImages function can be used to extract either or both types of the images from the response. Once an array of ScannedImage is obtained, we can iterate each ScannedImage and create an img element from it and then display images on the web page using function processScannedImage. Note that we use imagesScanned to track all ScannedImages so that we may manipulate later (e.g., upload to server).
Now, the scanned images are shown on the web page. To upload the images to the server side, please refer to the developer's guide.


Featured Clients
Sectors: Finance • Information Technoloy • Government • Healthcare • Industries • Education • (show all)